Cross-validation predictions¶
In addition to computing cross-validation scores, you can use cross-validation to produce predictions. Unlike traditional cross-validation, where folds are independent of one another, time-series folds may overlap (particularly in a sliding window). To account for this, folds that forecast the same time step average their forecasts using either a “mean” or “median” (tunable).
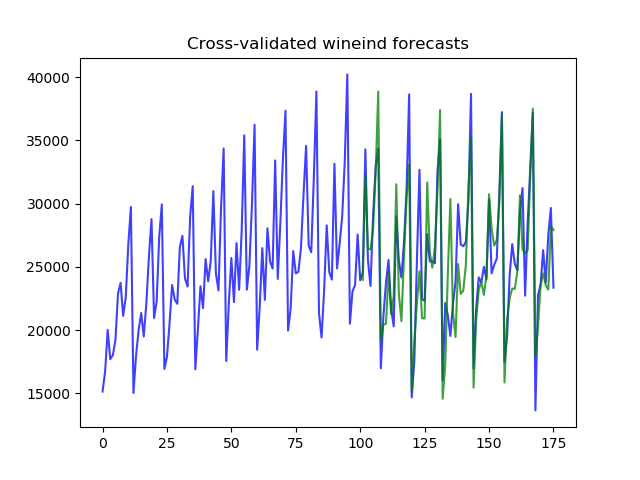
Out:
pmdarima version: 0.0.0
[CV] fold=0 ..........................................................
[CV] fold=1 ..........................................................
[CV] fold=2 ..........................................................
[CV] fold=3 ..........................................................
[CV] fold=4 ..........................................................
[CV] fold=5 ..........................................................
[CV] fold=6 ..........................................................
[CV] fold=7 ..........................................................
[CV] fold=8 ..........................................................
[CV] fold=9 ..........................................................
[CV] fold=10 .........................................................
[CV] fold=11 .........................................................
[CV] fold=12 .........................................................
[CV] fold=13 .........................................................
[CV] fold=14 .........................................................
[CV] fold=15 .........................................................
[CV] fold=16 .........................................................
[CV] fold=17 .........................................................
[CV] fold=18 .........................................................
[CV] fold=19 .........................................................
[CV] fold=20 .........................................................
[CV] fold=21 .........................................................
[CV] fold=22 .........................................................
[CV] fold=23 .........................................................
[CV] fold=24 .........................................................
[CV] fold=25 .........................................................
[CV] fold=26 .........................................................
[CV] fold=27 .........................................................
[CV] fold=28 .........................................................
[CV] fold=29 .........................................................
[CV] fold=30 .........................................................
[CV] fold=31 .........................................................
[CV] fold=32 .........................................................
[CV] fold=33 .........................................................
[CV] fold=34 .........................................................
[CV] fold=35 .........................................................
[CV] fold=36 .........................................................
[CV] fold=37 .........................................................
[CV] fold=38 .........................................................
[CV] fold=39 .........................................................
[CV] fold=40 .........................................................
[CV] fold=41 .........................................................
[CV] fold=42 .........................................................
[CV] fold=43 .........................................................
[CV] fold=44 .........................................................
[CV] fold=45 .........................................................
[CV] fold=46 .........................................................
[CV] fold=47 .........................................................
[CV] fold=48 .........................................................
[CV] fold=49 .........................................................
[CV] fold=50 .........................................................
[CV] fold=51 .........................................................
[CV] fold=52 .........................................................
[CV] fold=53 .........................................................
[CV] fold=54 .........................................................
[CV] fold=55 .........................................................
[CV] fold=56 .........................................................
[CV] fold=57 .........................................................
[CV] fold=58 .........................................................
[CV] fold=59 .........................................................
[CV] fold=60 .........................................................
[CV] fold=61 .........................................................
[CV] fold=62 .........................................................
[CV] fold=63 .........................................................
[CV] fold=64 .........................................................
[CV] fold=65 .........................................................
[CV] fold=66 .........................................................
[CV] fold=67 .........................................................
[CV] fold=68 .........................................................
[CV] fold=69 .........................................................
[CV] fold=70 .........................................................
[CV] fold=71 .........................................................
[CV] fold=72 .........................................................
print(__doc__)
# Author: Taylor Smith <taylor.smith@alkaline-ml.com>
import numpy as np
import pmdarima as pm
from pmdarima import model_selection
from matplotlib import pyplot as plt
print("pmdarima version: %s" % pm.__version__)
# Load the data and split it into separate pieces
y = pm.datasets.load_wineind()
est = pm.ARIMA(order=(1, 1, 2),
seasonal_order=(0, 1, 1, 12),
suppress_warnings=True)
cv = model_selection.SlidingWindowForecastCV(window_size=100, step=1, h=4)
predictions = model_selection.cross_val_predict(
est, y, cv=cv, verbose=2, averaging="median")
# plot the predictions over the original series
x_axis = np.arange(y.shape[0])
n_test = predictions.shape[0]
plt.plot(x_axis, y, alpha=0.75, c='b')
plt.plot(x_axis[-n_test:], predictions, alpha=0.75, c='g') # Forecasts
plt.title("Cross-validated wineind forecasts")
plt.show()
Total running time of the script: ( 2 minutes 41.260 seconds)